Mastering Asynchronous Programming in C#: From Beginner to Advanced
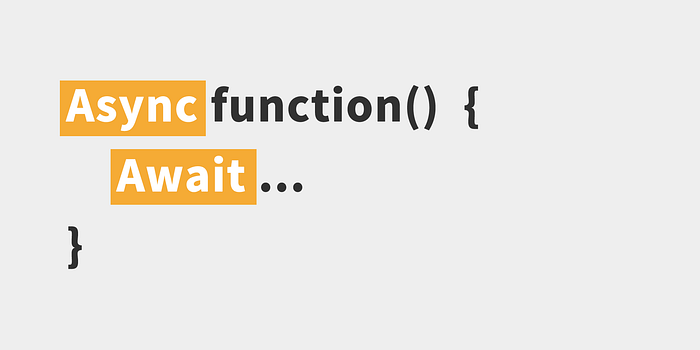
Asynchronous programming is a powerful feature in modern software development, allowing you to write code that can perform multiple tasks concurrently without blocking the main thread.
In C#, async
and await
are the cornerstone of this approach. Whether you’re new to C# or looking to refine your skills, this guide will take you through the essentials of asynchronous programming, from the basics to advanced techniques.
Understanding Asynchronous Programming
Before diving into async
and await
, let’s break down what asynchronous programming is and why it’s important. In simple terms, asynchronous programming allows your program to start a task and move on to another task before the first one is complete. This is particularly useful in scenarios where tasks involve waiting for external resources, such as reading files, querying a database, or making web requests.
Getting Started with async
and await
In C#, the async
keyword is used to declare a method as asynchronous. When you prefix a method with async
, you’re indicating that the method will contain one or more await
expressions. The await
keyword is used to pause the execution of an async
method until a task is complete, without blocking the calling thread.
Here’s a basic example:
public async Task<string> FetchDataAsync()
{
// Simulate a delay to represent a time-consuming operation
await Task.Delay(2000);
return "Data fetched";
}
In this example, FetchDataAsync
simulates an asynchronous operation using Task.Delay
to mimic a delay. The await
keyword tells the program to wait for the Task.Delay
to complete before continuing.
Calling Asynchronous Methods
To call an asynchronous method, use the await
keyword:
public async Task ProcessDataAsync()
{
string result = await FetchDataAsync();
Console.WriteLine(result);
}
Here, ProcessDataAsync
calls FetchDataAsync
and waits for it to complete before printing the result. Note that ProcessDataAsync
also needs to be marked with async
.
Error Handling in Asynchronous Methods
Handling errors in asynchronous methods is similar to synchronous code. Use try
and catch
blocks to manage exceptions:
public async Task ProcessDataAsync()
{
try
{
string result = await FetchDataAsync();
Console.WriteLine(result);
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
Intermediate Concepts: Synchronization and Cancellation
1. Task WhenAll and WhenAny
In scenarios where you need to run multiple asynchronous operations concurrently and wait for all of them to complete, use Task.WhenAll
:
public async Task ProcessMultipleTasksAsync()
{
Task task1 = FetchDataAsync();
Task task2 = FetchDataAsync();
await Task.WhenAll(task1, task2);
}
Alternatively, if you need to wait for any one of multiple tasks to complete, use Task.WhenAny
:
public async Task ProcessAnyTaskAsync()
{
Task task1 = FetchDataAsync();
Task task2 = FetchDataAsync();
Task completedTask = await Task.WhenAny(task1, task2);
// Handle completedTask here
}
2. Cancellation Tokens
To cancel an ongoing asynchronous operation, use CancellationToken
:
public async Task FetchDataWithCancellationAsync(CancellationToken cancellationToken)
{
try
{
await Task.Delay(5000, cancellationToken);
Console.WriteLine("Data fetched");
}
catch (OperationCanceledException)
{
Console.WriteLine("Operation was canceled");
}
}
Pass the CancellationToken
to tasks that support cancellation and handle OperationCanceledException
for proper cancellation logic.
Advanced Techniques: Optimization and Best Practices
1. Avoiding Blocking Calls
Always prefer asynchronous operations over blocking calls to avoid performance bottlenecks. For instance, avoid .Result
or .Wait()
on tasks, as they can lead to deadlocks or performance issues:
// Bad practice
var result = FetchDataAsync().Result;
// Good practice
var result = await FetchDataAsync();
2. Asynchronous Streams
With C# 8.0 and later, you can use asynchronous streams for processing data asynchronously. This is useful for scenarios like reading lines from a file or streaming data from a network source:
public async IAsyncEnumerable<string> ReadLinesAsync(string path)
{
using var stream = new StreamReader(path);
string line;
while ((line = await stream.ReadLineAsync()) != null)
{
yield return line;
}
}
You can iterate over asynchronous streams using await foreach
:
public async Task ProcessLinesAsync()
{
await foreach (var line in ReadLinesAsync("file.txt"))
{
Console.WriteLine(line);
}
}
3. Performance Considerations
Understand the trade-offs between asynchronous and synchronous code. Asynchronous programming can improve responsiveness and scalability but may introduce complexity. Measure and analyze performance to determine when and where to apply asynchronous patterns.
Conclusion
Asynchronous programming in C# with async
and await
is a fundamental skill for modern software development. By mastering these concepts, you can write more efficient, responsive, and scalable applications. Start with the basics, experiment with intermediate features, and explore advanced techniques to fully leverage the power of asynchronous programming in C#.